If you have a notification, which is only relevant for you when you are at home, it does not make sense to send it, if you are away. Instead, it would make sense to receive it the moment you get home.
To do this, simply add an Wait for a template
action before sending the notification, with the following content. This can be done via YAML configuration
- wait_template: "{{ is_state('person.nico', 'home') }}"
continue_on_timeout: true
or via the web interface
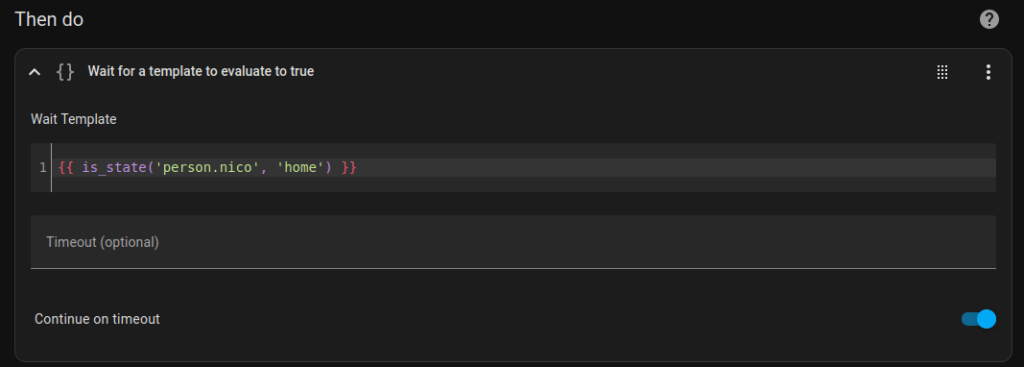
If you are currently at home, means the entity status is already in the state home
, it will be resolved immediately, otherwise it will wait until your status changes to home
.