const { executeHttpRequest } = require('@sap-cloud-sdk/http-client')
const FormData = require('form-data')
try {
//Create payload
const form = new FormData()
form.append('file', fileContent, {
contentType: 'application/pdf'
filename: 'test.pdf'
})
//Create headers
const headers = {
...form.getHeaders(),
'Content-Length': form.getLengthSync(),
}
//Send to Destination
const response = await executeHttpRequest(
{ destinationName: 'TESTINATION' },
{
method: 'POST',
url: 'myApiPath',
headers: headers,
data: form,
responseType: 'arraybuffer' // if you need the response data as buffer to prevent UTF-8 encoding
}
)
console.log({response})
} catch (error) {
console.error(error.message)
}
Month: July 2023
[Git] Branch Commands
https://git-scm.com/book/en/v2/Git-Branching-Basic-Branching-and-Merging
https://git-scm.com/book/en/v2/Git-Branching-Branch-Management
# create branch, switch to it and commit changes
git checkout -b hotfix
git commit -a -m 'Commit changes for fix'
# go back to main branch
git checkout main
# merge branch changes to main
git merge hotfix
# delete branch after merging, as it is not needed anymore
git branch -d hotfix
# check on which branch your are
git status
# list all branches including last commit on each branch
git branch -vv
# check which branches are already merged and which are not
git branch --merged
git branch --no-merged
# rename branche locally
git branch --move bad-branch-name corrected-branch-name
# push new name to github/gitlab
git push --set-upstream origin corrected-branch-name
# displays local and remote branches
git branch --all
# delete remote branch
git push origin --delete bad-branch-name
# push branch to remote
git push origin hotfix
[Android] Upgrading LineageOS 18.1 to 19.1 on my Xiaomi Mi 8 (dipper)
Install the Android Debug Bridge (ADB)
https://wiki.lineageos.org/adb_fastboot_guide.html
https://github.com/M0Rf30/android-udev-rules#installation
# check if device is found
adb devices
# reboot into sideload modus
adb reboot sideload
Or manually boot into TWRP recovery, holding Volume Up + Power when the phone is off. Navigate to Advanced
-> ADB Sideload
.
Update MIUI Firmware
Following the docs, I first had to check the Firmware version. V12.0.3.0.QEAMIXM was required, and I already had it installed.
If you’re on an older version, download the right MIUI Firmware for your device from https://xiaomifirmwareupdater.com/firmware/dipper/.
Flash the new Firmware via TWRP or via ADB sideload.
adb sideload fw_dipper_miui_MI8Global_V12.0.3.0.QEAMIXM_7619340f8c_10.0.zip
Download and flash new LineageOS image
I’m using the LineageOS fork LineageOS for microG. Download it from here: https://download.lineage.microg.org/dipper/ (MI 8 = dipper)
The upgrade steps are the same as for the official rom: https://wiki.lineageos.org/devices/dipper/upgrade. In my case only flashing the new image.
adb sideload lineage-19.1-20221217-microG-dipper.zip
[CAP] Fiori Elements – Add section with PDFViewer
I have a CAP Service that provides a PDF file that I needed to display in a Fiori Elements frontend using the sap.m.PDFViewer. The viewer should be placed in a section on the object page after navigating from the ListReport main page.
My CAP Service has the following annotations to provide the PDF.
entity pdfFiles : cuid, managed {
content : LargeBinary @stream @Core.MediaType: mediaType @Core.ContentDisposition.Filename: fileName @Core.ContentDisposition.Type: 'inline';
mediaType : String @Core.IsMediaType: true;
fileName : String @mandatory;
}
Add a custom section to your view following this example: https://sapui5.hana.ondemand.com/test-resources/sap/fe/core/fpmExplorer/index.html#/customElements/customSectionContent
Two steps are necessary.
1. Add a new section via the manifest. The template path should match your app namespace.
"ObjectPage": {
"type": "Component",
"id": "ObjectPage",
"name": "sap.fe.templates.ObjectPage",
"viewLevel": 1,
"options": {
"settings": {
"editableHeaderContent": false,
"entitySet": "pdfFiles",
"content": {
"body": {
"sections" : {
"myCustomSection": {
"template": "sap.fe.core.fpmExplorer.customSectionContent.CustomSection",
"title": "{i18n>pdfSection}",
"position": {
"placement": "After",
"anchor": "StandardSection"
}
}
}
}
}
}
}
}
2. Add the section content by defining a new fragment in the file CustomSection.fragment.xml
<core:FragmentDefinition xmlns:core="sap.ui.core" xmlns="sap.m" xmlns:l="sap.ui.layout" xmlns:macro="sap.fe.macros">
<ScrollContainer
height="100%"
width="100%"
horizontal="true"
vertical="true">
<FlexBox direction="Column" renderType="Div" class="sapUiSmallMargin">
<PDFViewer source="{content}" title="{fileName}" height="1200px">
<layoutData>
<FlexItemData growFactor="1" />
</layoutData>
</PDFViewer>
</FlexBox>
</ScrollContainer>
</core:FragmentDefinition>
On the ObjectPage you will now have a new section containing the PDFViewer.
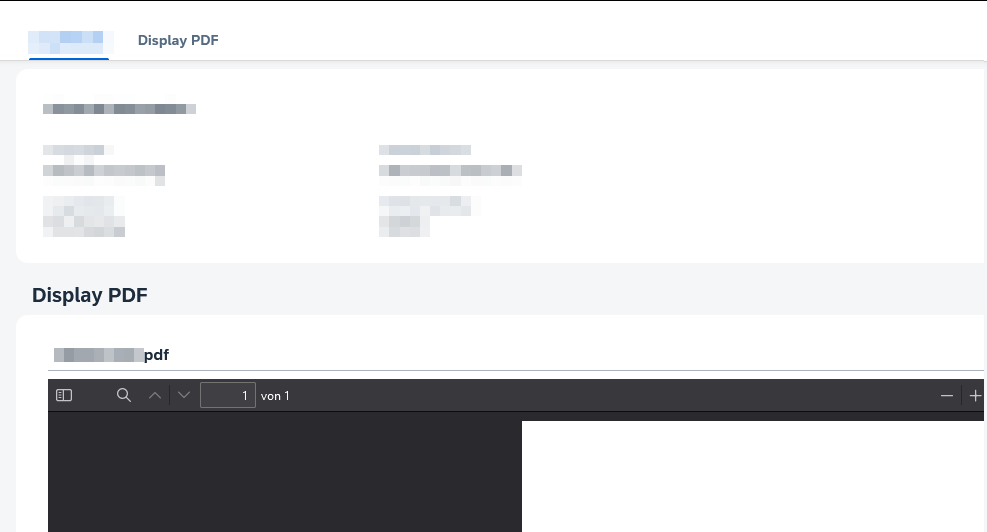
[CAP] Fiori Elements – Display managed fields as value help
data-model.cds
using {
managed
} from '@sap/cds/common';
entity managedEntity: managed {
key ID : UUID;
field : String;
}
annotations.cds
using myService as service from '../../srv/myService';
annotate service.managedEntity with @(
Capabilities.SearchRestrictions: {Searchable: false},
UI.PresentationVariant : {
SortOrder : [{
Property : createdAt,
Descending: true
}],
Visualizations: ['@UI.LineItem']
},
UI.HeaderInfo : {
TypeName : '{i18n>myEntity}',
TypeNamePlural: '{i18n>myEntities}',
},
UI.SelectionFields : [
createdAt,
createdBy
],
UI.LineItem : [
]
) {
createdAt @UI.HiddenFilter : false;
createdBy @UI.HiddenFilter : false;
};