I have a CAP Service that provides a PDF file that I needed to display in a Fiori Elements frontend using the sap.m.PDFViewer. The viewer should be placed in a section on the object page after navigating from the ListReport main page.
My CAP Service has the following annotations to provide the PDF.
entity pdfFiles : cuid, managed {
content : LargeBinary @stream @Core.MediaType: mediaType @Core.ContentDisposition.Filename: fileName @Core.ContentDisposition.Type: 'inline';
mediaType : String @Core.IsMediaType: true;
fileName : String @mandatory;
}
Add a custom section to your view following this example: https://sapui5.hana.ondemand.com/test-resources/sap/fe/core/fpmExplorer/index.html#/customElements/customSectionContent
Two steps are necessary.
1. Add a new section via the manifest. The template path should match your app namespace.
"ObjectPage": {
"type": "Component",
"id": "ObjectPage",
"name": "sap.fe.templates.ObjectPage",
"viewLevel": 1,
"options": {
"settings": {
"editableHeaderContent": false,
"entitySet": "pdfFiles",
"content": {
"body": {
"sections" : {
"myCustomSection": {
"template": "sap.fe.core.fpmExplorer.customSectionContent.CustomSection",
"title": "{i18n>pdfSection}",
"position": {
"placement": "After",
"anchor": "StandardSection"
}
}
}
}
}
}
}
}
2. Add the section content by defining a new fragment in the file CustomSection.fragment.xml
<core:FragmentDefinition xmlns:core="sap.ui.core" xmlns="sap.m" xmlns:l="sap.ui.layout" xmlns:macro="sap.fe.macros">
<ScrollContainer
height="100%"
width="100%"
horizontal="true"
vertical="true">
<FlexBox direction="Column" renderType="Div" class="sapUiSmallMargin">
<PDFViewer source="{content}" title="{fileName}" height="1200px">
<layoutData>
<FlexItemData growFactor="1" />
</layoutData>
</PDFViewer>
</FlexBox>
</ScrollContainer>
</core:FragmentDefinition>
On the ObjectPage you will now have a new section containing the PDFViewer.
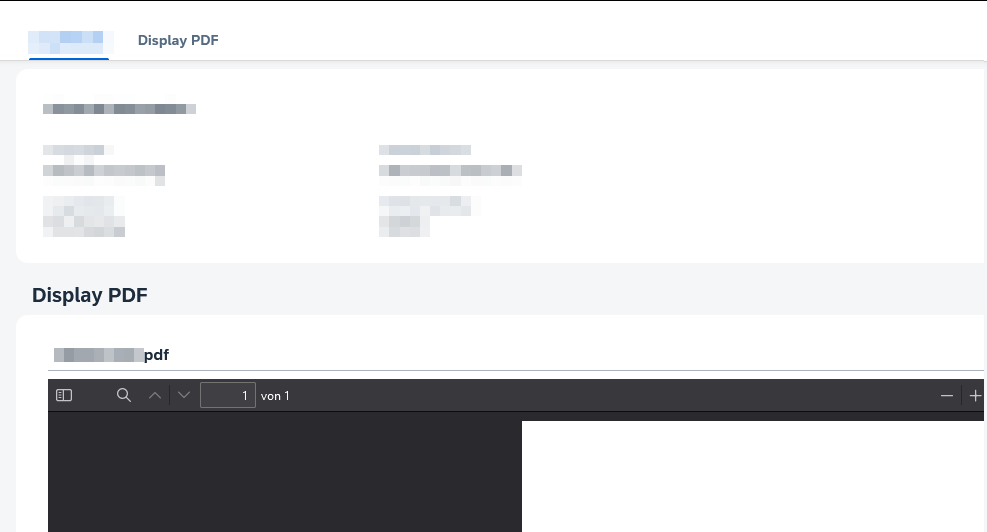