cl_http_ext_webapp=>create_url_for_bsp_application( EXPORTING bsp_application = bsp_application " Name der BSP Applikation
bsp_start_page = bsp_start_page " Startseite der BSP Applikation
bsp_start_parameters = bsp_start_parameters " Startparameter der BSP Applikation
IMPORTING abs_url = DATA(lv_absolute_url ). " Absolute URL (Protokol, Host, Port, ...) der BSP Applikation
Category: ABAP
ABAP
[ABAP] Display PDF in HTML Control
DATA lt_data TYPE STANDARD TABLE OF x255.
CALL FUNCTION 'SCMS_XSTRING_TO_BINARY'
EXPORTING
buffer = lv_xstring "pdf data
TABLES
binary_tab = lt_data.
DATA(o_html) = NEW cl_gui_html_viewer( parent = cl_gui_container=>default_screen ).
* URL zu HTML holen
DATA: lv_url TYPE swk_url.
o_html->load_data( EXPORTING type = 'BIN'
subtype = 'PDF'
IMPORTING assigned_url = lv_url
CHANGING data_table = lt_data ).
* HTML anzeigen
o_html->show_url( lv_url ).
* erzwingt Anzeige über cl_gui_container=>default_screen
WRITE: / space.
[ABAP] OData – Request Parameter
You can access the request parameter from anywhere in the *_DPC_EXT class via:
mr_request_details->parameters
[ABAP] Convert table of strings to a single string
DATA(lv_string) = REDUCE #( INIT str = || FOR line IN table_of_strings NEXT str = str && line).
[ABAP] Create ToC for a given transport, release it and download it as ZIP
Related Reports:
https://nocin.eu/abap-download-transport-as-zip/
https://nocin.eu/abap-import-transport-from-zip/
*&---------------------------------------------------------------------*
*& Report Z_ZIP_TOC
*&---------------------------------------------------------------------*
*& For the givin transport, this report creates a transport of copies (ToC),
*& releases it, and then download the ToC as ZIP file to your filesystem.
*& The given request can be in sate unreleased!
*&---------------------------------------------------------------------*
REPORT Z_ZIP_TOC.
INITIALIZATION.
SELECTION-SCREEN BEGIN OF BLOCK bl01 WITH FRAME TITLE TEXT-t01.
PARAMETERS p_trkorr LIKE e070-trkorr OBLIGATORY.
PARAMETERS p_ttext TYPE as4text.
SELECTION-SCREEN END OF BLOCK bl01.
SELECTION-SCREEN BEGIN OF BLOCK bl02 WITH FRAME TITLE TEXT-t02.
PARAMETERS p_sapdir TYPE string LOWER CASE OBLIGATORY DEFAULT '/usr/sap/trans/'.
PARAMETERS p_lcldir TYPE string LOWER CASE OBLIGATORY DEFAULT 'C:\temp\'.
SELECTION-SCREEN END OF BLOCK bl02.
AT SELECTION-SCREEN ON VALUE-REQUEST FOR p_trkorr.
CALL FUNCTION 'TR_F4_REQUESTS'
EXPORTING
iv_trkorr_pattern = p_trkorr
IMPORTING
ev_selected_request = p_trkorr.
START-OF-SELECTION.
" Read description of provided transport
DATA ls_request TYPE trwbo_request.
CALL FUNCTION 'TR_READ_REQUEST'
EXPORTING
iv_read_e07t = 'X'
iv_trkorr = p_trkorr
CHANGING
cs_request = ls_request
EXCEPTIONS
error_occured = 1
no_authorization = 2
OTHERS = 3.
IF sy-subrc <> 0.
MESSAGE 'Could not read Transport description' TYPE 'E'.
ENDIF.
" Read all objects for provided transport
DATA: lt_objects TYPE tr_objects,
lt_keys TYPE tr_keys.
CALL FUNCTION 'TR_GET_OBJECTS_OF_REQ_AN_TASKS'
EXPORTING
is_request_header = VALUE trwbo_request_header( trkorr = p_trkorr )
iv_condense_objectlist = 'X'
IMPORTING
et_objects = lt_objects
et_keys = lt_keys
EXCEPTIONS
invalid_input = 1
OTHERS = 2.
IF sy-subrc <> 0.
MESSAGE 'Could not read Transport objects' TYPE 'E'.
ENDIF.
" Create new ToC
DATA ls_request_header TYPE trwbo_request_header.
CALL FUNCTION 'TR_INSERT_REQUEST_WITH_TASKS'
EXPORTING
iv_type = 'T'
iv_text = COND as4text( WHEN p_ttext IS INITIAL THEN ls_request-h-as4text
ELSE p_ttext )
iv_owner = sy-uname
iv_target = 'DUM'
IMPORTING
es_request_header = ls_request_header
EXCEPTIONS
insert_failed = 1
enqueue_failed = 2
OTHERS = 3.
IF sy-subrc <> 0.
MESSAGE 'Transport creation failed' TYPE 'E'.
ENDIF.
DATA(lv_trkorr_toc) = ls_request_header-trkorr.
" Add all object to the new ToC
CALL FUNCTION 'TRINT_APPEND_COMM'
EXPORTING
wi_exclusive = 'X'
wi_sel_e071 = 'X'
wi_sel_e071k = 'X'
wi_trkorr = lv_trkorr_toc
TABLES
wt_e071 = lt_objects
wt_e071k = lt_keys
EXCEPTIONS
e071k_append_error = 1
e071_append_error = 2
trkorr_empty = 3
OTHERS = 4.
IF sy-subrc <> 0.
MESSAGE 'Could not append objects to ToC' TYPE 'E'.
ENDIF.
" Release ToC Transport
DATA lt_messages TYPE ctsgerrmsgs.
CALL FUNCTION 'TRINT_RELEASE_REQUEST'
EXPORTING
iv_trkorr = lv_trkorr_toc
* IV_DIALOG = 'X'
* IV_AS_BACKGROUND_JOB = ' '
* IV_SUCCESS_MESSAGE = 'X'
* IV_WITHOUT_OBJECTS_CHECK = ' '
* IV_CALLED_BY_ADT = ' '
* IV_CALLED_BY_PERFORCE = ' '
* IV_WITHOUT_DOCU = ' '
iv_without_locking = 'X'
* IV_DISPLAY_EXPORT_LOG = 'X'
* IV_IGNORE_WARNINGS = ' '
* IV_SIMULATION = ' '
IMPORTING
et_messages = lt_messages
EXCEPTIONS
cts_initialization_failure = 1
enqueue_failed = 2
no_authorization = 3
invalid_request = 4
request_already_released = 5
repeat_too_early = 6
object_lock_error = 7
object_check_error = 8
docu_missing = 9
db_access_error = 10
action_aborted_by_user = 11
export_failed = 12
execute_objects_check = 13
release_in_bg_mode = 14
release_in_bg_mode_w_objchk = 15
error_in_export_methods = 16
object_lang_error = 17
OTHERS = 18.
IF sy-subrc <> 0.
MESSAGE 'Could not release ToC' TYPE 'E'.
cl_demo_output=>display( lt_messages ).
ENDIF.
" Download released ToC as ZIP
DATA lv_xcontent_k TYPE xstring.
DATA lv_xcontent_r TYPE xstring.
DATA(lv_transdir_k) = |{ p_sapdir }cofiles/K{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|.
DATA(lv_transdir_r) = |{ p_sapdir }data/R{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|.
TRY.
" K
OPEN DATASET lv_transdir_k FOR INPUT IN BINARY MODE.
READ DATASET lv_transdir_k INTO lv_xcontent_k.
CLOSE DATASET lv_transdir_k.
" R
OPEN DATASET lv_transdir_r FOR INPUT IN BINARY MODE.
READ DATASET lv_transdir_r INTO lv_xcontent_r.
CLOSE DATASET lv_transdir_r.
" Add to ZIP
DATA(lo_zipper) = NEW cl_abap_zip( ).
lo_zipper->add( name = |K{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|
content = lv_xcontent_k ).
lo_zipper->add( name = |R{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|
content = lv_xcontent_r ).
" Download ZIP
DATA(lv_xzip) = lo_zipper->save( ).
" Convert to raw data
DATA(lt_data) = cl_bcs_convert=>xstring_to_solix( iv_xstring = lv_xzip ).
" Set zip filename
DATA(lv_zip_name) = COND #( WHEN p_ttext IS INITIAL THEN |{ ls_request-h-as4text }_{ lv_trkorr_toc }|
ELSE |{ p_ttext }_{ lv_trkorr_toc }| ).
" Replace every character that is not [a-zA-Z0-9_] with '_'.
REPLACE ALL OCCURRENCES OF REGEX '[^\w]+' IN lv_zip_name WITH '_'.
cl_gui_frontend_services=>gui_download( EXPORTING filename = p_lcldir && lv_zip_name && '.zip'
filetype = 'BIN'
CHANGING data_tab = lt_data ).
CATCH cx_root INTO DATA(e_text).
MESSAGE e_text->get_text( ) TYPE 'E'.
ENDTRY.
MESSAGE |{ lv_zip_name }.zip created and downloaded to { p_lcldir }| TYPE 'S'.
[ABAP] ALV traffic lights
TYPES: BEGIN OF ty_log,
status TYPE icon-id,
END OF ty_log.
DATA gt_log TYPE TABLE OF ty_log.
gt_log = VALUE #( ( status = icon_red_light )
( status = icon_yellow_light )
( status = icon_green_light ) ).
TRY.
cl_salv_table=>factory( IMPORTING r_salv_table = DATA(alv_table)
CHANGING t_table = gt_log ).
alv_table->display( ).
CATCH cx_salv_msg.
ENDTRY.
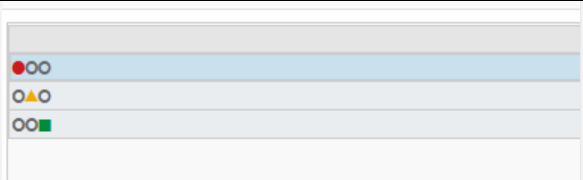
icon_red
, icon_yellow
etc. will be automatically loaded from the TYPE-POOL: icon
during runtime.
You can check all available icons via the table icon
.
SELECT SINGLE id INTO @DATA(icon_red) FROM icon WHERE name = 'ICON_RED_LIGHT'.
[ABAP] Download Transport as ZIP
Related Reports:
https://nocin.eu/abap-import-transport-from-zip/
https://nocin.eu/abap-create-toc-for-a-given-transport-release-it-and-download-it-as-zip/
This report can be handy, especially since S/4HANA 2023 seems to have restricted the “classic” import way by using TCode CG3Y and CG3Z. See note 1949906, where it is recommended to create a custom report.
*&---------------------------------------------------------------------*
*& Report ZIP_TRANSPORT
*&---------------------------------------------------------------------*
*&
*&---------------------------------------------------------------------*
REPORT zip_transport.
SELECTION-SCREEN BEGIN OF BLOCK bl01 WITH FRAME TITLE TEXT-t01.
PARAMETERS p_trkorr LIKE e070-trkorr OBLIGATORY.
PARAMETERS p_ttext TYPE as4text.
SELECTION-SCREEN END OF BLOCK bl01.
SELECTION-SCREEN BEGIN OF BLOCK bl02 WITH FRAME TITLE TEXT-t02.
PARAMETERS p_sapdir TYPE string LOWER CASE OBLIGATORY DEFAULT '/usr/sap/trans/'.
PARAMETERS p_lcldir TYPE string LOWER CASE OBLIGATORY DEFAULT 'C:\temp\'.
SELECTION-SCREEN END OF BLOCK bl02.
START-OF-SELECTION.
" Check if Transport is released
DATA ls_request TYPE trwbo_request.
CALL FUNCTION 'TR_READ_REQUEST'
EXPORTING
iv_read_e070 = abap_true
iv_read_e07t = abap_true
iv_trkorr = p_trkorr
CHANGING
cs_request = ls_request
EXCEPTIONS
error_occured = 1
no_authorization = 2
OTHERS = 3.
IF ls_request-h-trstatus <> 'R'.
MESSAGE 'Transport not yet released' TYPE 'E'.
ENDIF.
" Read released Transport
DATA lv_xcontent_k TYPE xstring.
DATA lv_xcontent_r TYPE xstring.
DATA(lv_transdir_k) = |{ p_sapdir }cofiles/K{ p_trkorr+4 }.{ p_trkorr(3) }|.
DATA(lv_transdir_r) = |{ p_sapdir }data/R{ p_trkorr+4 }.{ p_trkorr(3) }|.
TRY.
" K
OPEN DATASET lv_transdir_k FOR INPUT IN BINARY MODE.
READ DATASET lv_transdir_k INTO lv_xcontent_k.
CLOSE DATASET lv_transdir_k.
" R
OPEN DATASET lv_transdir_r FOR INPUT IN BINARY MODE.
READ DATASET lv_transdir_r INTO lv_xcontent_r.
CLOSE DATASET lv_transdir_r.
" Add to ZIP
DATA(lo_zipper) = NEW cl_abap_zip( ).
lo_zipper->add( name = |K{ p_trkorr+4 }.{ p_trkorr(3) }|
content = lv_xcontent_k ).
lo_zipper->add( name = |R{ p_trkorr+4 }.{ p_trkorr(3) }|
content = lv_xcontent_r ).
" Download ZIP
DATA(lv_xzip) = lo_zipper->save( ).
" Convert to raw data
DATA(lt_data) = cl_bcs_convert=>xstring_to_solix( iv_xstring = lv_xzip ).
" Set zip filename
DATA(lv_zip_name) = COND #( WHEN p_ttext IS INITIAL THEN |{ ls_request-h-as4text }_{ p_trkorr }|
ELSE |{ p_ttext }_{ p_trkorr }| ).
" Replace every character that is not [a-zA-Z0-9_] with '_'.
REPLACE ALL OCCURRENCES OF REGEX '[^\w]+' IN lv_zip_name WITH '_'.
cl_gui_frontend_services=>gui_download( EXPORTING filename = p_lcldir && lv_zip_name && '.zip'
filetype = 'BIN'
CHANGING data_tab = lt_data ).
CATCH cx_root INTO DATA(e_text).
MESSAGE e_text->get_text( ) TYPE 'E'.
ENDTRY.
MESSAGE |{ lv_zip_name }.zip created and downloaded to { p_lcldir }| TYPE 'S'.
[ABAP] Sum column using reduce with where condition
SELECT * FROM sflight INTO TABLE @DATA(lt_sflight).
DATA(a319_paymant_sum) = REDUCE #( INIT sum = 0
FOR flight IN lt_sflight WHERE ( planetype = 'A319' )
NEXT sum += flight-paymentsum ).
[ABAP] Finding maximum value of specific column in itab
DATA(maximum) = REDUCE #( INIT max = VALUE myType( )
FOR line IN myTable
NEXT max = COND #( WHEN line-myColumn > max THEN line-myColumn
ELSE max ) ).
[ABAP] OData – GET_STREAM implementation to return a PDF
METHOD /iwbep/if_mgw_appl_srv_runtime~get_stream.
* This method get's called when a media file is queried with $value. A binary stream will be returned.
TRY.
DATA(file_id) = VALUE zfile_id( it_key_tab[ name = 'file_id' ]-value ).
CATCH cx_sy_itab_line_not_found.
RETURN. " leave here when no file_id provided
ENDTRY.
DATA(ls_file) = get_file( file_id ) " read your file you want to return (if it's not yet a binary stream, convert it)
DATA(ls_stream) = VALUE ty_s_media_resource( value = ls_file-value
mime_type = ls_file-mimetype ). " in my case it's 'application/pdf'
" necessary to display the filename instead of $value in the viewer title
TRY.
" create pdf object
DATA(lo_fp) = cl_fp=>get_reference( ).
DATA(lo_pdfobj) = lo_fp->create_pdf_object( connection = 'ADC' ).
lo_pdfobj->set_document( pdfdata = ls_stream-value ).
" set title
lo_pdfobj->set_metadata( VALUE #( title = ls_file-filename ) ).
lo_pdfobj->execute( ).
" get pdf with title
lo_pdfobj->get_document( IMPORTING pdfdata = ls_stream-value ).
CATCH cx_fp_runtime_internal
cx_fp_runtime_system
cx_fp_runtime_usage INTO DATA(lo_fpex).
ENDTRY.
copy_data_to_ref( EXPORTING is_data = ls_stream
CHANGING cr_data = er_stream ).
" necessary for the pdf to be opened inline instead of a download (also sets the filename when downloaded)
/iwbep/if_mgw_conv_srv_runtime~set_header( VALUE #( name = 'content-disposition'
value = |inline; filename={ ls_file-filename }| ) ).
ENDMETHOD
Quick way to open a PDFViewer in your UI5 App:
const pdfViewer = new PDFViewer()
pdfViewer.setSource("/sap/opu/odata/ZMY_SEVICE" + my_path + "/$value") // my_path could be something like this "/PdfSet('file_id')"
pdfViewer.setTitle("My PDFViewer Title") // title of the popup, not the viewer
pdfViewer.open()