Every few months, I run into this issue when adding a torrent to transgui:
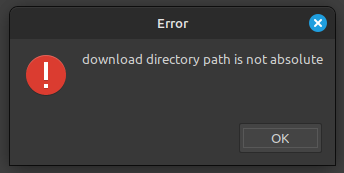
Although there is a 5-year-old closed issue on this bug, which also led to a code adjustment, this bug still seems to exist: https://github.com/transmission-remote-gui/transgui/issues/1270
The user Kethsar has probably already found the right cause and gives some hints on how to solve it. At least it helped me to find a workaround:
- close transgui if it’s running, otherwise your changes will get overwritten again
- nano ~/.config/Transmission\ Remote\ GUI/transgui.ini
- search for the
[AddTorrent.transmission]
section - remove some entries which a related, e.g.
Folder1=/my/path/1
FolHit1=1
FolExt1=
LastDt1=18.02.2024
Folder2=/my/path/2
FolHit2=3
FolExt2=
LastDt2=18.02.2024
...
- save & quit nano
- start trangui again and try to add a torrent again
It seems like the issues occurs, when the [AddTorrent.transmission]
section reaches Folder50
.