const start = new Date()
const end = new Date()
start.setHours(0, 0, 0, 0)
end.setHours(23, 59, 59, 999)
console.log(start.toISOString())
console.log(end.toISOString())
Category: JavaScript / nodejs
[nodejs] base64 to buffer and vice versa
const myBase64File = "JVBERi0xLjQKJcOkw7zDtsOfCjIgMCBvYmoKPDwvTGVuZ3....."
const buffer = Buffer.from(myBase64File, "base64")
const base64 = buffer.toString("base64")
[nodejs] iterate through fetch response headers
https://github.github.io/fetch/#Headers
const response = await fetch("https://example.com/api")
for (const [key, value] of response.headers) {
console.log(key, value)
}
An alternative would be forEach()
response.headers.forEach((value, key) => {
console.log(value, key)
})
Or using the entries()
iterator (ES8)
const headerIterator = response.headers.entries()
console.log(headerIterator.next().value)
console.log(headerIterator.next().value)
To add a new header just use set()
response.set(key, value)
[JavaScript] Get the Last Item in an Array
const animals = [‘cat’, ‘dog’, ‘horse’]
// before
const lastItem = animals[animals.length - 1]
// ES2022
const lastItem = animals.at(-1)
[JavaScript] Download base64 encoded file within a browser
const sBase64 = "JVBERi0xLjQKJcOkw7zDtsOfCjIgMCBvYmoKPDwvTGVuZ3....."
const arrayBuffer = new Uint8Array([...window.atob(sBase64)].map(char => char.charCodeAt(0)))
const fileLink = document.createElement('a')
fileLink.href = window.URL.createObjectURL(new Blob([arrayBuffer]))
fileLink.setAttribute('download', "example.pdf")
document.body.appendChild(fileLink)
fileLink.click()
Or use the npm package FileSaver.
import { saveAs } from "file-saver";
const sBase64 = "JVBERi0xLjQKJcOkw7zDtsOfCjIgMCBvYmoKPDwvTGVuZ3....."
const buffer = Buffer.from(sBase64, "base64") //Buffer is only available when using nodejs
saveAs(new Blob([buffer]), "example.pdf")
[JavaScript] map an array of objects to return an array of objects
Recently I hat to create an array of objects from another array of objects to change some property names and to enrich it with some values. To iterate over the array I used the map()
function. But when trying to return the new object using the brackets {}
, the map function instead expects me trying to write a function… Therefore you need to wrap the return object in ()
const aPersons = [{
id: 1,
name: 'max'
}, {
id: 2,
name: 'peter'
}]
const aResult = aPersons.map(person => ({ value: person.id, text: person.name, someboolean: true }))
console.log(aResult)
[Postman] Visualize base64 image
If you have a service which returns a payload like the following (including a base64 encoded jpeg) you can display it directly in postman.
{
"photo": "/9j/4AAQSkZJRgABAgAAAQABAAD/2wBDAAMCAgMCAgMDAwMEAwMEBQgFBQQEBQoHBwYIDAoMDAsK\r\nCwsND..............",
"photoId": "192",
"mimeType": "image/jpeg"
}
This can be done with a few lines of code. In Postman navigate to the “Tests” tab:

and insert the following lines:
//output to postman console
console.log("PhotoId: " + pm.response.json()["photoId"]);
console.log("Base64: " + pm.response.json()["photo"]);
//output in visualize tab
let template = `<img src='{{img}}'/>`;
pm.visualizer.set(template, {
img: `data:image/jpeg;base64,${pm.response.json()["photo"]}`
});
In the “Visualize” tab you should now find your image
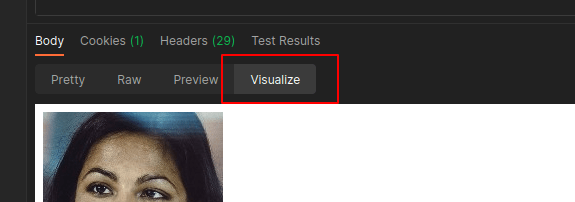
[PDF.js] Prevent loading default pdf
This can be done by appending ?file=
to the src path, like it is mentionend here.
<!-- "?file=" prevents loading the default document -->
<iframe id="pdf-js-viewer" src="/pdf/web/viewer.html?file=" title="webviewer" frameborder="0" width="100%" height="700" allowfullscreen="" webkitallowfullscreen=""/>
Or by setting the variable defaultUrl
to blank using the onload event.
document.addEventListener("webviewerloaded", async () => {
let pdfViewerIFrame = document.getElementById("pdf-js-viewer")
//https://github.com/mozilla/pdf.js/blob/master/web/app_options.js
pdfViewerIFrame.contentWindow.PDFViewerApplicationOptions.set("defaultUrl", "") //prevent loading default pdf
})
[nodejs] read and write a file
https://nodejs.dev/learn/reading-files-with-nodejs
https://nodejs.dev/learn/writing-files-with-nodejs
const fs = require("fs")
try {
// read from local folder
const localPDF = fs.readFileSync('PDFs/myFile.pdf')
//write back to local folder
fs.writeFileSync('PDFs/writtenBack.pdf', localPDF )
} catch (err) {
console.error(err)
}
Converting to Base64
try {
// read from local folder
const localPDF = fs.readFileSync('PDFs/myFile.pdf')
const localBase64 = localPDF.toString('base64')
//write back to local folder
fs.writeFileSync(`PDFs/writtenBack.pdf`, localBase64, {encoding: 'base64'})
} catch (err) {
console.error(err)
}
Reading and writing using streams with pipe
//read and write local file
const reader = fs.createReadStream("PDFs/myFile.pdf")
const writer = fs.createWriteStream('PDFs/writtenBack.pdf');
reader.pipe(writer)