The Attribute ACTION_COMMENTS is of type SWC_VALUE, which is a char with length 255.
If the entered text has less than < 255 characters, there is just one element named ‘ACTION_COMMENTS’ in the workitem container.

If the user entered a text with more than 255 characters, the text is split and there are more elements named with ‘ACTION_COMMENTS_1’, ‘ACTION_COMMENTS_2’ etc. (Note 3017539)

There is the function module SAP_WAPI_READ_CONTAINER to read Workitems. As input for the function module, you need the workitemId from the decision step. You will then find the ACTION_COMMENTS in the simple_container table.
DATA: return_code TYPE sy-subrc,
simple_container TYPE TABLE OF swr_cont,
message_lines TYPE TABLE OF swr_messag,
message_struct TYPE TABLE OF swr_mstruc,
subcontainer_bor_objects TYPE TABLE OF swr_cont,
subcontainer_all_objects TYPE TABLE OF swr_cont,
object_content TYPE TABLE OF solisti1.
" Read the work item container from the work item ID
CALL FUNCTION 'SAP_WAPI_READ_CONTAINER'
EXPORTING
workitem_id = wiid
IMPORTING
return_code = return_code
TABLES
simple_container = simple_container
message_lines = message_lines
message_struct = message_struct
subcontainer_bor_objects = subcontainer_bor_objects
subcontainer_all_objects = subcontainer_all_objects.
TRY.
DATA(text) = simple_container[ element = 'ACTION_COMMENTS' ]-value.
CATCH cx_sy_itab_line_not_found.
" Check for ACTION_COMMENTS_1 etc.
" or follow the approach below
ENDTRY.
The comment is also added as attachment to the workitem. Just check the table subcontainer_all_objects, which is also returned by the previous function module, for attribute _ATTACH_COMMENT_OBJECTS (or _ATTACH_OBJECT or DECISION_NOTE). With function module SO_DOCUMENT_READ_API1 you can then get the actual comment.
" Read the _ATTACH_COMMENT_OBJECTS element
" There can be more than one comment, just take the last one
LOOP AT subcontainer_all_objects INTO DATA(comment_object) WHERE element = '_ATTACH_COMMENT_OBJECTS'.
ENDLOOP.
CHECK comment_object-value IS NOT INITIAL.
" Read the SOFM Document
CALL FUNCTION 'SO_DOCUMENT_READ_API1'
EXPORTING
document_id = CONV so_entryid( comment_object-value )
TABLES
object_content = object_content
EXCEPTIONS
OTHERS = 1.
LOOP AT object_content INTO DATA(lv_soli).
CONCATENATE text lv_soli-line INTO text.
ENDLOOP.
As you get a table as a result, it’s properly easier to read long comments this way.
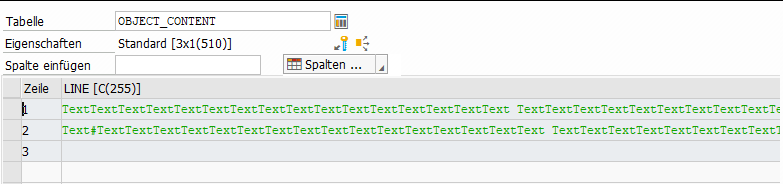