Bei einer Abwesenheit wie z.B. Urlaub, die über eine Monatsgrenze oder über einige Feiertage hinweg geht, kann es hilfreich sein, die konkreten Abtragungen einzusehen, um zu verstehen, welche Tage in dem Zeitraum denn wirklich Urlaub waren.
Dazu einfach die Abwesenheit öffnen in der PA20 und auf Springen → Abtragungen (Shift+F8) gehen.

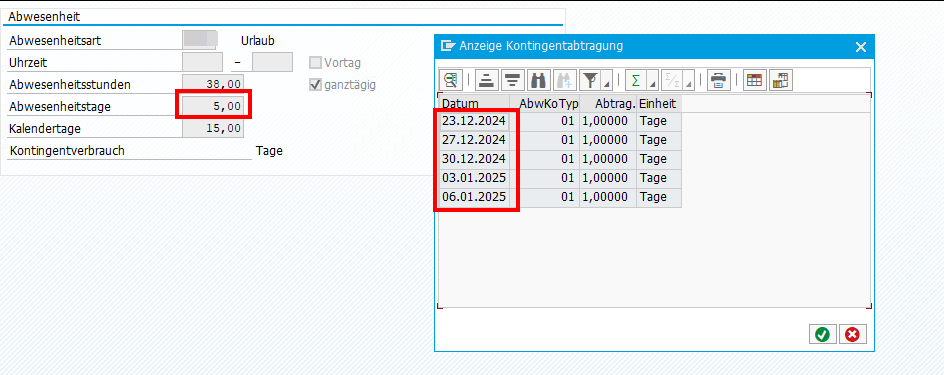
Man kann die Kontingentabtragung auch für das komplette Kontingent eines Jahres einsehen via IT2006.
Die zugehörige Datenbanktabelle ist PTQUODED
. Um die Abträge für eine bestimmte Abwesenheitsart zu selektieren, muss noch ein JOIN auf die PA2001 über die DOCNR gemacht werden. Zumindest ist das der einzige Weg, den ich herausfinden konnte. 🙂
SELECT SUM( a~quode )
FROM ptquoded AS a
JOIN pa2001 AS b
ON a~pernr = b~pernr
AND a~docnr = b~docnr
INTO DATA(urlaubsabtrag)
WHERE a~pernr = pernr-pernr
AND a~datum BETWEEN pn-begda AND pn-endda
AND b~subty = 0100. " Abwesenheitsart, welche Selektiert werden soll