const { executeHttpRequest } = require('@sap-cloud-sdk/http-client')
const FormData = require('form-data')
try {
//Create payload
const form = new FormData()
form.append('file', fileContent, {
contentType: 'application/pdf'
filename: 'test.pdf'
})
//Create headers
const headers = {
...form.getHeaders(),
'Content-Length': form.getLengthSync(),
}
//Send to Destination
const response = await executeHttpRequest(
{ destinationName: 'TESTINATION' },
{
method: 'POST',
url: 'myApiPath',
headers: headers,
data: form,
responseType: 'arraybuffer' // if you need the response data as buffer to prevent UTF-8 encoding
}
)
console.log({response})
} catch (error) {
console.error(error.message)
}
Tag: SAP
[CAP] Fiori Elements – Add section with PDFViewer
I have a CAP Service that provides a PDF file that I needed to display in a Fiori Elements frontend using the sap.m.PDFViewer. The viewer should be placed in a section on the object page after navigating from the ListReport main page.
My CAP Service has the following annotations to provide the PDF.
entity pdfFiles : cuid, managed {
content : LargeBinary @stream @Core.MediaType: mediaType @Core.ContentDisposition.Filename: fileName @Core.ContentDisposition.Type: 'inline';
mediaType : String @Core.IsMediaType: true;
fileName : String @mandatory;
}
Add a custom section to your view following this example: https://sapui5.hana.ondemand.com/test-resources/sap/fe/core/fpmExplorer/index.html#/customElements/customSectionContent
Two steps are necessary.
1. Add a new section via the manifest. The template path should match your app namespace.
"ObjectPage": {
"type": "Component",
"id": "ObjectPage",
"name": "sap.fe.templates.ObjectPage",
"viewLevel": 1,
"options": {
"settings": {
"editableHeaderContent": false,
"entitySet": "pdfFiles",
"content": {
"body": {
"sections" : {
"myCustomSection": {
"template": "sap.fe.core.fpmExplorer.customSectionContent.CustomSection",
"title": "{i18n>pdfSection}",
"position": {
"placement": "After",
"anchor": "StandardSection"
}
}
}
}
}
}
}
}
2. Add the section content by defining a new fragment in the file CustomSection.fragment.xml
<core:FragmentDefinition xmlns:core="sap.ui.core" xmlns="sap.m" xmlns:l="sap.ui.layout" xmlns:macro="sap.fe.macros">
<ScrollContainer
height="100%"
width="100%"
horizontal="true"
vertical="true">
<FlexBox direction="Column" renderType="Div" class="sapUiSmallMargin">
<PDFViewer source="{content}" title="{fileName}" height="1200px">
<layoutData>
<FlexItemData growFactor="1" />
</layoutData>
</PDFViewer>
</FlexBox>
</ScrollContainer>
</core:FragmentDefinition>
On the ObjectPage you will now have a new section containing the PDFViewer.
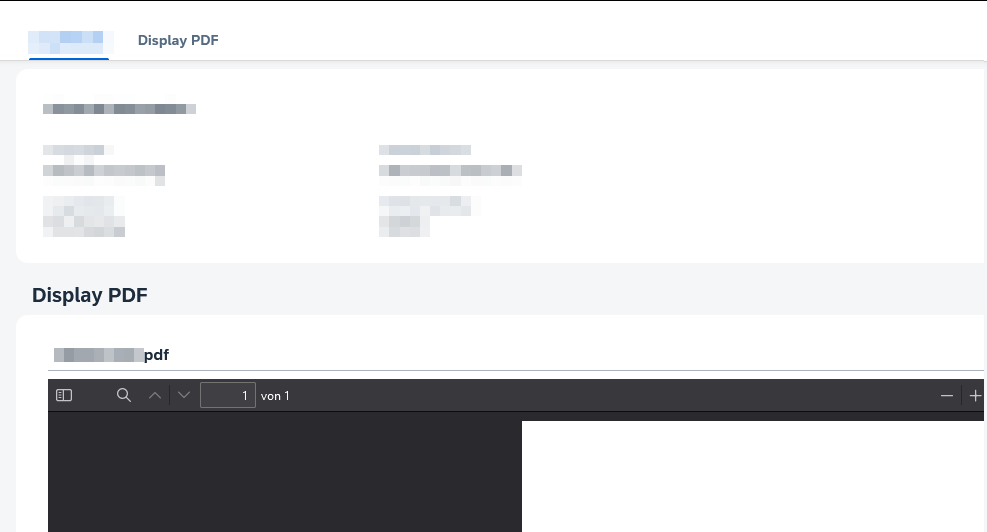
[CAP] Fiori Elements – Display managed fields as value help
data-model.cds
using {
managed
} from '@sap/cds/common';
entity managedEntity: managed {
key ID : UUID;
field : String;
}
annotations.cds
using myService as service from '../../srv/myService';
annotate service.managedEntity with @(
Capabilities.SearchRestrictions: {Searchable: false},
UI.PresentationVariant : {
SortOrder : [{
Property : createdAt,
Descending: true
}],
Visualizations: ['@UI.LineItem']
},
UI.HeaderInfo : {
TypeName : '{i18n>myEntity}',
TypeNamePlural: '{i18n>myEntities}',
},
UI.SelectionFields : [
createdAt,
createdBy
],
UI.LineItem : [
]
) {
createdAt @UI.HiddenFilter : false;
createdBy @UI.HiddenFilter : false;
};
[SAP] Login to SAP for Me and other SAP Websites via Certificate
Go to https://me.sap.com/app/sappassport, request your certificate and install it in your Browser. Working with the BTP is much more convenient now, as you don’t have to enter your credentials all the time.
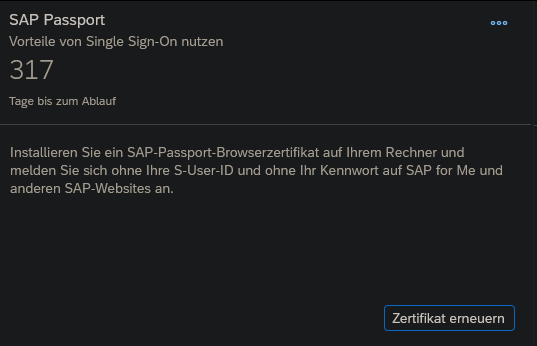
[CAP] CQL Expand Composition / Deep read
In the documentation they always use a star in string literals like this o => o.`*`
to select all fields, but when running this it always failed. However, after some tests I found that this format works with brackets o => o.('*')
https://cap.cloud.sap/docs/guides/providing-services/#–-deep-read
entity myEntity: cuid, managed {
field1 : String;
comp : Composition of one myComposition;
}
aspect myComposition: cuid, managed {
myCompField: String;
}
const result = await SELECT.from(myEntity)
.columns(e => {
e('*')
e.comp(c => c.myCompField) //expand composition, but only select 'myCompField'
})
Update 14.09.2023: is now fixed (#)
[CAP] CQL Deep Update
A Composition of one
can be updated via the Parent Entity, like we would do it, when dealing with an Association to one
.
entity myEntity: cuid, managed {
field1 : String;
comp : Composition of one myComposition;
}
aspect myComposition: cuid, managed {
myCompField: String;
}
const { myEntity } = srv.entities
const EntityComposition = srv.entities['myEntity.myComposition'] // composition
srv.after('CREATE', EntityComposition , async (comp, req) => {
await UPDATE(myEntity, comp.ID)
.set({
comp : [{ myCompField: 'test' }]
})
})
A Composition of many
must be updated via the actual Composition Entity.
entity myEntity: cuid, managed {
field1 : String;
comp : Composition to many myComposition;
}
aspect myComposition: cuid, managed {
myCompField: String;
}
const EntityComposition = srv.entities['myEntity.myComposition'] // composition
srv.after('CREATE', EntityComposition , async (comp, req) => {
await UPDATE(EntityComposition, { up__ID: comp.up__ID, ID: comp.ID }).set({ myCompField: 'test' })
})
[CAP] UploadSet http test file
How to create an uploadSet in combination with a CAP backend: https://blogs.sap.com/2021/08/18/my-journey-towards-using-ui5-uploadset-with-cap-backend/
You can test the uploadSet CAP backend part using these http calls:
### Create file
# @name file
POST http://localhost:4004/v2/admin/Files
Authorization: Basic admin:
Content-Type: application/json
{
"mediaType": "image/png",
"fileName": "picture.png",
"size": 1000
}
### Fill Variable from Response
@ID = {{file.response.body.$.d.ID}}
### Upload Binary PNG content
PUT http://localhost:4004/v2/admin/Files({{ID}})/content
Authorization: Basic admin:
Content-Type: image/png
< ./picture.png
### Get uploaded png
GET http://localhost:4004/v2/admin/Files({{ID}})/content
Authorization: Basic admin:
The picture.png file must be in the same folder as the http test file.
[CAP] Dynamically set destination in package.json for an external connection
"cds": {
"requires": {
"sfsf": {
"kind": "odata-v2",
"credentials": {
"destination": "<set during runtime>",
"path": "/odata/v2",
"requestTimeout": 18000000
}
}
}
},
/*
* Handover query to some external SF OData Service to fecth the requested data
*/
srv.on("READ", Whatever, async req => {
const sf_api_def = cds.env.requires['sfsf'] //defined in package.json
sf_api_def.credentials.destination = "myDestinationName" //set your Destination name, could come from a customizing table
const sfsfSrv = await cds.connect.to(sf_api_def)
return await sfsfSrv.run(req.query)
})
[CAP] Providing destinations locally using .env
The provided example
destinations="[{\"name\": \"TESTINATION\", \"url\": \"http://url.hana.ondemand.com\", \"username\": \"DUMMY_USER\", \"password\": \"EXAMPLE_PASSWORD\"}]"
didn’t work for me. I had to change it to the following format:
destinations=[{"name": "TESTINATION", "url": "http://url.hana.ondemand.com", "username": "DUMMY_USER", "password": "EXAMPLE_PASSWORD"}]
You can check the current value using:
process.env.destinations
[ABAP] Create ToC for a given transport, release it and download it as ZIP
Related Reports:
https://nocin.eu/abap-download-transport-as-zip/
https://nocin.eu/abap-import-transport-from-zip/
*&---------------------------------------------------------------------*
*& Report Z_ZIP_TOC
*&---------------------------------------------------------------------*
*& For the givin transport, this report creates a transport of copies (ToC),
*& releases it, and then download the ToC as ZIP file to your filesystem.
*& The given request can be in sate unreleased!
*&---------------------------------------------------------------------*
REPORT Z_ZIP_TOC.
INITIALIZATION.
SELECTION-SCREEN BEGIN OF BLOCK bl01 WITH FRAME TITLE TEXT-t01.
PARAMETERS p_trkorr LIKE e070-trkorr OBLIGATORY.
PARAMETERS p_ttext TYPE as4text.
SELECTION-SCREEN END OF BLOCK bl01.
SELECTION-SCREEN BEGIN OF BLOCK bl02 WITH FRAME TITLE TEXT-t02.
PARAMETERS p_sapdir TYPE string LOWER CASE OBLIGATORY DEFAULT '/usr/sap/trans/'.
PARAMETERS p_lcldir TYPE string LOWER CASE OBLIGATORY DEFAULT 'C:\temp\'.
SELECTION-SCREEN END OF BLOCK bl02.
AT SELECTION-SCREEN ON VALUE-REQUEST FOR p_trkorr.
CALL FUNCTION 'TR_F4_REQUESTS'
EXPORTING
iv_trkorr_pattern = p_trkorr
IMPORTING
ev_selected_request = p_trkorr.
START-OF-SELECTION.
" Read description of provided transport
DATA ls_request TYPE trwbo_request.
CALL FUNCTION 'TR_READ_REQUEST'
EXPORTING
iv_read_e07t = 'X'
iv_trkorr = p_trkorr
CHANGING
cs_request = ls_request
EXCEPTIONS
error_occured = 1
no_authorization = 2
OTHERS = 3.
IF sy-subrc <> 0.
MESSAGE 'Could not read Transport description' TYPE 'E'.
ENDIF.
" Read all objects for provided transport
DATA: lt_objects TYPE tr_objects,
lt_keys TYPE tr_keys.
CALL FUNCTION 'TR_GET_OBJECTS_OF_REQ_AN_TASKS'
EXPORTING
is_request_header = VALUE trwbo_request_header( trkorr = p_trkorr )
iv_condense_objectlist = 'X'
IMPORTING
et_objects = lt_objects
et_keys = lt_keys
EXCEPTIONS
invalid_input = 1
OTHERS = 2.
IF sy-subrc <> 0.
MESSAGE 'Could not read Transport objects' TYPE 'E'.
ENDIF.
" Create new ToC
DATA ls_request_header TYPE trwbo_request_header.
CALL FUNCTION 'TR_INSERT_REQUEST_WITH_TASKS'
EXPORTING
iv_type = 'T'
iv_text = COND as4text( WHEN p_ttext IS INITIAL THEN ls_request-h-as4text
ELSE p_ttext )
iv_owner = sy-uname
iv_target = 'DUM'
IMPORTING
es_request_header = ls_request_header
EXCEPTIONS
insert_failed = 1
enqueue_failed = 2
OTHERS = 3.
IF sy-subrc <> 0.
MESSAGE 'Transport creation failed' TYPE 'E'.
ENDIF.
DATA(lv_trkorr_toc) = ls_request_header-trkorr.
" Add all object to the new ToC
CALL FUNCTION 'TRINT_APPEND_COMM'
EXPORTING
wi_exclusive = 'X'
wi_sel_e071 = 'X'
wi_sel_e071k = 'X'
wi_trkorr = lv_trkorr_toc
TABLES
wt_e071 = lt_objects
wt_e071k = lt_keys
EXCEPTIONS
e071k_append_error = 1
e071_append_error = 2
trkorr_empty = 3
OTHERS = 4.
IF sy-subrc <> 0.
MESSAGE 'Could not append objects to ToC' TYPE 'E'.
ENDIF.
" Release ToC Transport
DATA lt_messages TYPE ctsgerrmsgs.
CALL FUNCTION 'TRINT_RELEASE_REQUEST'
EXPORTING
iv_trkorr = lv_trkorr_toc
* IV_DIALOG = 'X'
* IV_AS_BACKGROUND_JOB = ' '
* IV_SUCCESS_MESSAGE = 'X'
* IV_WITHOUT_OBJECTS_CHECK = ' '
* IV_CALLED_BY_ADT = ' '
* IV_CALLED_BY_PERFORCE = ' '
* IV_WITHOUT_DOCU = ' '
iv_without_locking = 'X'
* IV_DISPLAY_EXPORT_LOG = 'X'
* IV_IGNORE_WARNINGS = ' '
* IV_SIMULATION = ' '
IMPORTING
et_messages = lt_messages
EXCEPTIONS
cts_initialization_failure = 1
enqueue_failed = 2
no_authorization = 3
invalid_request = 4
request_already_released = 5
repeat_too_early = 6
object_lock_error = 7
object_check_error = 8
docu_missing = 9
db_access_error = 10
action_aborted_by_user = 11
export_failed = 12
execute_objects_check = 13
release_in_bg_mode = 14
release_in_bg_mode_w_objchk = 15
error_in_export_methods = 16
object_lang_error = 17
OTHERS = 18.
IF sy-subrc <> 0.
MESSAGE 'Could not release ToC' TYPE 'E'.
cl_demo_output=>display( lt_messages ).
ENDIF.
" Download released ToC as ZIP
DATA lv_xcontent_k TYPE xstring.
DATA lv_xcontent_r TYPE xstring.
DATA(lv_transdir_k) = |{ p_sapdir }cofiles/K{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|.
DATA(lv_transdir_r) = |{ p_sapdir }data/R{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|.
TRY.
" K
OPEN DATASET lv_transdir_k FOR INPUT IN BINARY MODE.
READ DATASET lv_transdir_k INTO lv_xcontent_k.
CLOSE DATASET lv_transdir_k.
" R
OPEN DATASET lv_transdir_r FOR INPUT IN BINARY MODE.
READ DATASET lv_transdir_r INTO lv_xcontent_r.
CLOSE DATASET lv_transdir_r.
" Add to ZIP
DATA(lo_zipper) = NEW cl_abap_zip( ).
lo_zipper->add( name = |K{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|
content = lv_xcontent_k ).
lo_zipper->add( name = |R{ lv_trkorr_toc+4 }.{ lv_trkorr_toc(3) }|
content = lv_xcontent_r ).
" Download ZIP
DATA(lv_xzip) = lo_zipper->save( ).
" Convert to raw data
DATA(lt_data) = cl_bcs_convert=>xstring_to_solix( iv_xstring = lv_xzip ).
" Set zip filename
DATA(lv_zip_name) = COND #( WHEN p_ttext IS INITIAL THEN |{ ls_request-h-as4text }_{ lv_trkorr_toc }|
ELSE |{ p_ttext }_{ lv_trkorr_toc }| ).
" Replace every character that is not [a-zA-Z0-9_] with '_'.
REPLACE ALL OCCURRENCES OF REGEX '[^\w]+' IN lv_zip_name WITH '_'.
cl_gui_frontend_services=>gui_download( EXPORTING filename = p_lcldir && lv_zip_name && '.zip'
filetype = 'BIN'
CHANGING data_tab = lt_data ).
CATCH cx_root INTO DATA(e_text).
MESSAGE e_text->get_text( ) TYPE 'E'.
ENDTRY.
MESSAGE |{ lv_zip_name }.zip created and downloaded to { p_lcldir }| TYPE 'S'.