Here are the notes I took while investigating SAP’s relatively ‘new’ overtime apps.
SAP Fiori for SAP Business Suite
– My Overtime Requests (Fiori 2.0)
– Approve Overtime Requests (Fiori 2.0)
SAP S/4HANA
– My Overtime Requests (Fiori 2.0)
– Approve Overtime Requests (Fiori 2.0)
SAP Fiori Apps Reference Library
– F4939
– F4937
Component:
– PA_FIO_OVT
Packages:
– PAOC_TIM_OVERTIMEQTA_REQ_CORE (Mein Mehrarbeitskontingent: Core-Funktionen)
– PAOC_TIM_OVERTIMEQTA_REQ_UIA (UIA = User Interface Adapter)
– PAOC_TM_TIMEDATE_REQ_CORE (Zeitdatenanforderung: Core-Funktionen)
– ODATA_HCMFAB_MYOVERTIMEQUOTA
– HCM_FAB_MYOVERTIMEQUOTA (contains BSP HCMFAB_OT_MAN)
– HCM_FAB_APPROVE_OVERTIME (contains BSP HCMFAB_OTQ_APR)
Reports
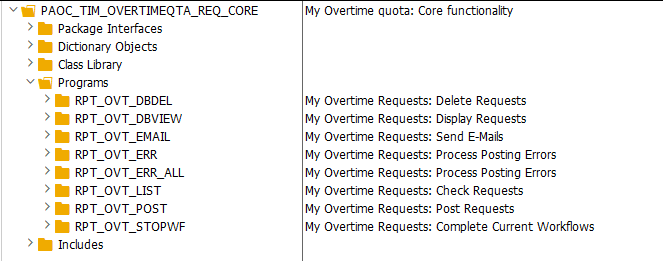
It is recommended to run the reports RPT_OVT_POST and RPT_OVT_EMAIL as a job.
Using RPT_OVT_UIA_TEST, you can simply simulate Overtime Requests in the backend to test your customizing.
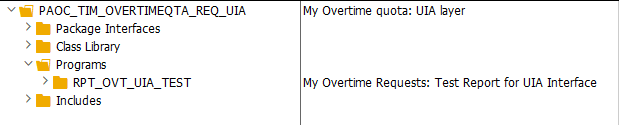
OData
– HCMFAB_MYOVERTIMEQUOTA_SRV (service name)
– HCMFAB_MYOVERTIMEQUOTA (SEGW Project)
Workflow
– WS02400057 (PT_OVTREQ)
Cluster Tables
– PTREQ_HEADER
– PTREQ_ITEMS
– PTREQ_ACTOR
– PTREQ_NOTICE
“On Behalf of” is supported
– 2806560
Customizing
SPRO → Personnel Management → Employee Self-Service (Web Dynpro ABAP) → Service-Specific Settings → Working Time → My Overtime Requests.
Adjusting the Fiori UI
If you want to hide fields in the Fiori frontend, or you want to adjust some labels, you can simply do this via Customizing:
My Overtime Requests → Layout of the Web Application → Define Field Selection
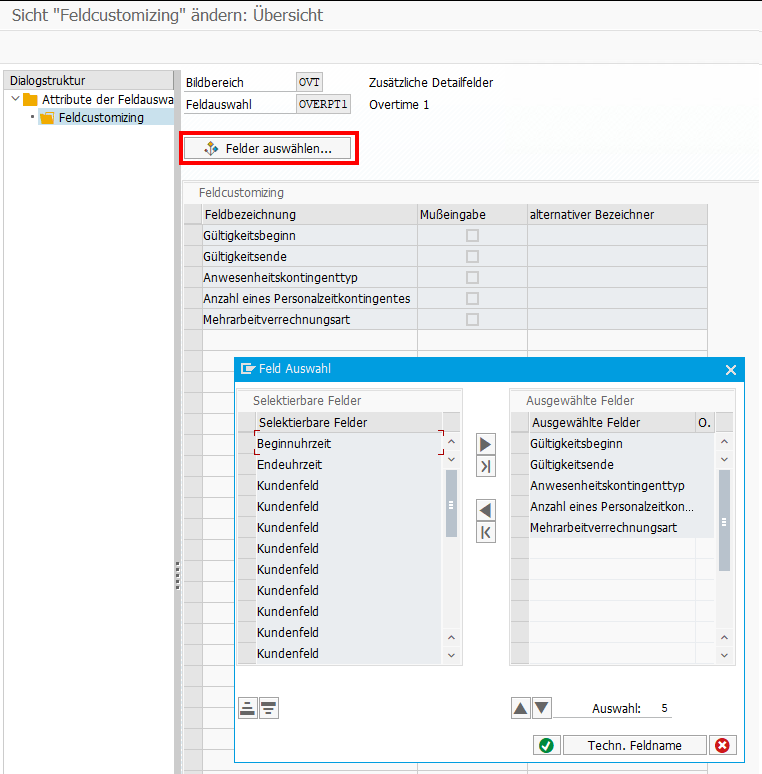
Multi-Level Approval for Time Data Requests (HRPT_B_TMD_MULTI_APPROVERS)
The BAdI is called when opening the My Overtime Request App and a second time when clicking on the New
Button to create a new OVT request. It’s not triggered when sending the request, what I initially expected.
To reuse the code from the sample class,
* "Determine approver level
* DATA(cust_key_request_fields) = VALUE cl_hrpt_ovt_const=>ovt_cust_key_request_fields( pernr = iv_pernr
* ktart = iv_subtype
* date = is_request_period-start_date ).
* TRY.
* DATA(approval_settings) = cl_hrpt_ovt_cust_factory=>get_customizing_reader( )->get_approval_settings( REF #( cust_key_request_fields ) ).
* CATCH cx_hrpt_tmd.
* RETURN.
* ENDTRY.
* IF approval_settings-use_multiple_approver = abap_false.
* RETURN.
* ENDIF.
....
* "If no last approvers exist, use line manager, HR BP and HR Admin by default
* CALL FUNCTION 'BAPI_GET_LINE_MANAGER'
* EXPORTING
* im_objid = iv_pernr
* IMPORTING
* es_approver = line_manager
* ev_has_manager = approver_exists.
* IF approver_exists = abap_true.
* last_seqnr = last_seqnr + 1.
* line_manager-seqnr = last_seqnr.
* APPEND line_manager TO rt_approver_tab.
* IF lines( rt_approver_tab ) >= approval_settings-approver_level.
* RETURN.
* ENDIF.
* ENDIF.
you have to set the amount of approval steps in the customizing.
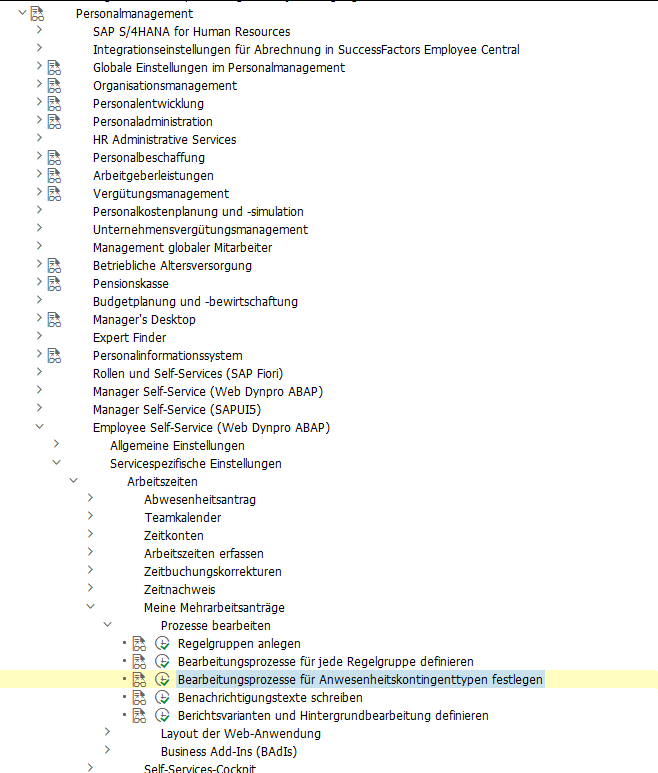
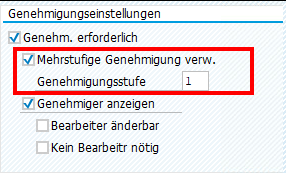
Custom Workflow
If you want to replace the default Workflow WS02400057 (PT_OVTREQ) with a custom one, you must adjust the customizing (as described here for the standard WF), and you’ll need to create a new BAdI Implementation for HCMFAB_MYOTQ_APPROVAL_INBOX
analog HCMFAB_MYOTQ_APPROVAL_INBOX
to get the Approve and Rejects Buttons working by providing the Workflow-ID and decision Step-ID.
Extensibility
Although it should be possible to extend both Fiori Apps via Adaptation Projects, I was unable to do so. Either it’s a problem with the new adaption editor, which got new released while writing the blog, or it is a problem with the apps itself. Whatever I tried, I was always running in issues.
Therefore, I did it the “classic” way and created an Extension Project and used the provided Extension Points. For the Approval App, I was unable to preview the application directly in BAS, perhaps because it is only an Inbox integration and does not run standalone. I therefore always had to deploy, to test my changes.