Recently, I had the task of assigning a workflow decision step to some users that were customized in a Z-Table. Normally I would have done this, by creating a container element “Actors”, a task that calls a method to return these Actors and use these Actors in the decision step. But this time I tried a custom AC Rule for the first time and I must say it was much easier than expected.
To begin, simply create a function module. The function module only needs two table parameters, AC_CONTAINER
and ACTOR_TAB
. They can be copied from RH_GET_ACTORS or GM_GET_RESP_FROM_INTERNAL. There you can also check, how to access the AC_CONTAINER
table, if you need any input values from the WF. In my case, I just had to read the Z-Table and return the result via ACTOR_TAB
.
SELECT * FROM ztable INTO TABLE @DATA(lt_table).
LOOP at lt_table INTO DATA(ls_table).
"you can also check if the provided user exists by using function module 'SUSR_USER_CHECK_EXISTENCE'
APPEND VALUE #( otype = 'US' objid = ls_table-userid ) TO actor_tab.
ENDLOOP.
IF lines( actor_tab) = 0.
RAISE nobody_found.
ENDIF.
Then simply go to tcode PFAC, create a rule, provide the function module and check the flag box at the end.

In the PFAC transaction, you can also simulate the rule resolution.
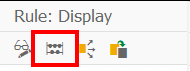
If everything works fine, add the new created rule to the decision step.
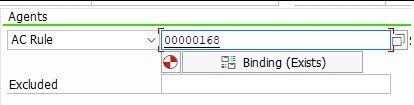
And done. Really straight forward.