using my.Model as db from '../db/data-model';
service myService @(requires: 'authenticated-user') {
@readonly
@cds.odata.valuelist
entity uniqueValues as select from db.Table distinct {
key field
};
}
Year: 2023
[CAP] Update standalone approuter
You can simply update your standalone approuter using cf push
,
cd ~/projects/bookshop/app/approuter/
cf push bookshop-approuter # take your approuter name from the mta.yaml file
cd ~/projects/bookshop/
[nodejs] workspaces
If you have subdirectories or additional applications which have its own package.json file, you can add them via the workspaces setting to your main project.
{
"name": "my-project",
"workspaces": [
"./app/*"
]
}
When running npm install
it will now also install the dependencies of all projects in the app folder.
[SAP] Set default download path in SU01
SU01 -> Edit user -> Parameter -> Add Parameter GR8 and your preferred path -> Save
[SAP] Reset different SAP buffers
https://wiki.scn.sap.com/wiki/display/Basis/How+to+Reset+different+SAP+buffers
/$SYNC - Resets the buffers of the application server
/$CUA - Resets the CUA buffer of the application server
/$TAB - Resets the TABLE buffers of the application server
/$NAM - Resets the nametab buffer of the application server
/$DYN - Resets the screen buffer of the application server
/$ESM - Resets the Exp./ Imp. Shared Memory Buffer of the application server
/$PXA - Resets the Program (PXA) Buffer of the application server.
/$OBJ - Resets the Shared Buffer of the application server.
[ABAP] convert string to xstring and convert back
DATA(lv_string) = |My string I want to convert to xstring.|.
TRY.
DATA(lv_xstring) = cl_abap_codepage=>convert_to( lv_string ).
DATA(lv_string_decoded) = cl_abap_codepage=>convert_from( lv_xstring ).
WRITE: / lv_string,
/ lv_xstring,
/ lv_string_decoded.
CATCH cx_root INTO DATA(e).
WRITE: / e->get_text( ).
ENDTRY.
[ABAP] Filter table using VALUE FOR
DATA(lt_result) = VALUE z_type( FOR line IN lt_table
WHERE ( value IN lr_values )
( field = line-value ) ).
[ABAP] Selection Screen Tabbed Block
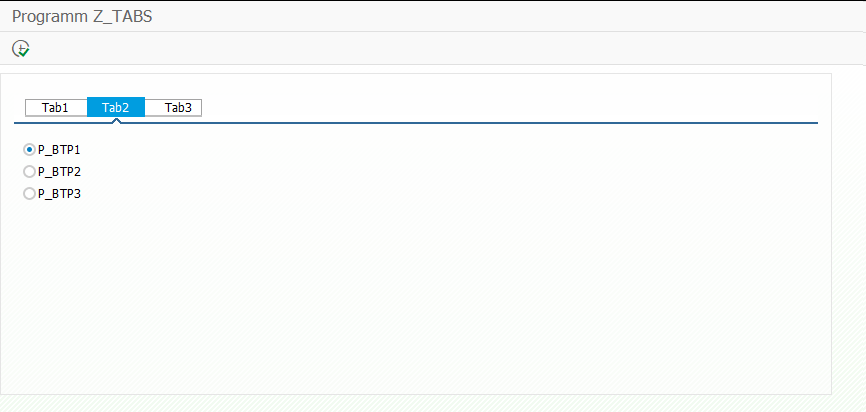
SELECTION-SCREEN BEGIN OF BLOCK bl4 WITH FRAME TITLE TEXT-t01.
SELECTION-SCREEN BEGIN OF TABBED BLOCK tbl FOR 10 LINES.
SELECTION-SCREEN TAB (15) tbl_tab1 USER-COMMAND tab1 DEFAULT SCREEN 1001.
SELECTION-SCREEN TAB (15) tbl_tab2 USER-COMMAND tab2 DEFAULT SCREEN 1002.
SELECTION-SCREEN TAB (15) tbl_tab3 USER-COMMAND tab3 DEFAULT SCREEN 1003.
SELECTION-SCREEN END OF BLOCK tbl.
SELECTION-SCREEN END OF BLOCK bl4.
* Subscreen 1001 Tab1
SELECTION-SCREEN BEGIN OF SCREEN 1001 AS SUBSCREEN.
PARAMETERS p_bool1 TYPE abap_bool AS CHECKBOX.
SELECTION-SCREEN END OF SCREEN 1001.
* Subscreen 1002 Tab2
SELECTION-SCREEN BEGIN OF SCREEN 1002 AS SUBSCREEN.
PARAMETERS p_btp1 TYPE flag RADIOBUTTON GROUP rbg DEFAULT 'X'.
PARAMETERS p_btp2 TYPE flag RADIOBUTTON GROUP rbg.
PARAMETERS p_btp3 TYPE flag RADIOBUTTON GROUP rbg.
SELECTION-SCREEN END OF SCREEN 1002.
* Subscreen 1003 Tab3
SELECTION-SCREEN BEGIN OF SCREEN 1003 AS SUBSCREEN.
PARAMETERS p_bool2 TYPE flag AS CHECKBOX.
SELECTION-SCREEN END OF SCREEN 1003.
INITIALIZATION.
" provide tab names
tbl_tab1 = 'Tab1'.
tbl_tab2 = 'Tab2'.
tbl_tab3 = 'Tab3'.
" set active tab (activetab value must be in uppercase)
tbl-activetab = 'TAB2'.
tbl-dynnr = 1002.
tbl-prog = sy-repid.
AT SELECTION-SCREEN.
" click on tabstrip event
CASE sy-ucomm.
WHEN 'TAB1'.
MESSAGE 'TAB1' TYPE 'S'.
WHEN 'TAB2'.
MESSAGE 'TAB2' TYPE 'S'.
WHEN 'TAB3'.
MESSAGE 'TAB3' TYPE 'S'.
ENDCASE.
[ABAP] Replace unusual characters with ordinary characters in a String
DATA(unusual) = 'á Ă é Ä Ö Ü ä ö ü ß'.
DATA(pretty) = VALUE string( ).
CALL FUNCTION 'SCP_REPLACE_STRANGE_CHARS'
EXPORTING
intext = unusual
IMPORTING
outtext = pretty.
WRITE / unusual.
WRITE / pretty. "a A e Ae Oe Ue ae oe ue ss
[CAP] min and max functions
Since there is nothing in the official CAP documentation about min and max functions, I figured out the following syntax:
const result1 = await cds.run(`SELECT *, MAX(seqNr) FROM ${myTable} LIMIT 1`) //returns array
const result2 = await SELECT.one.from(myTable, [`MAX(seqNr)`]).columns('*') //returns object
const result3 = await SELECT.one.from(myTable).columns('MAX(seqNr)') //returns object containing only the max counter value